Devlog 4: Back to Square 1!
Welcome to Week 01 of Production!
Our project showed promise and was accepted by our supervisors! That means we’re now entering full production. Last Tuesday, we kicked off a fresh project, same idea, but built properly this time.
Since this week was all about setting up the foundation, the technical section will be a bit shorter than usual. We haven't added new mechanics yet, but we’ve reimplemented what we had correctly based on what we learned from the prototype.
That said, the artists could finally show of their skills this tim, so let’s dive in!
ART
Hey yall! Laurens here, like afformentioned, the artists did indeed get to get going this week!
I have been working on the guns and getting those ready for action, the first plan was to do all of them this week, but it was decided that a few would get priority to get a base going before we continue to the rest.
(Here is the example of our AK, tis but a humble low poly model, but we genuinly don't really need more detail since from our camera distance you can not see more of it anyways.)
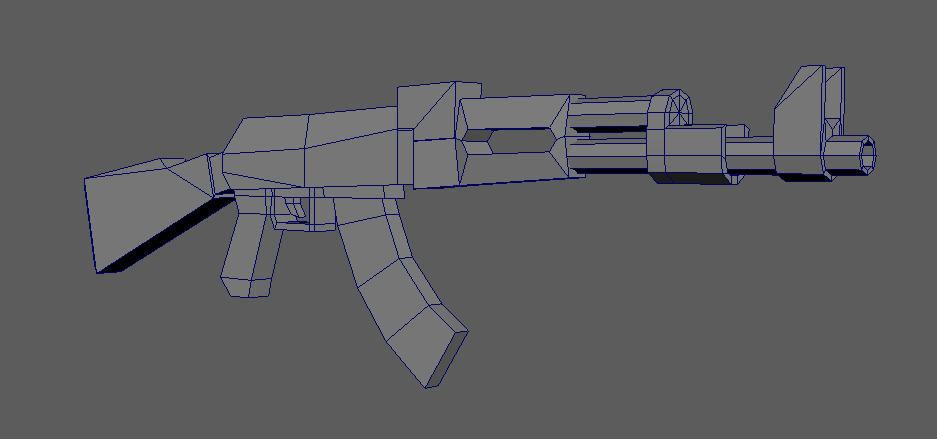
Since I now had time over this week I started on the character design, which, after confirmation from the coders and the other artists, can be turned into a 3D model by hopefully next week.
The original idea was 4 different characters, but this idea was canned until we have time left at the end of production, which is very fair.
So, I decided to try to combine all 4! The skater girl, the baseball player, the engineer and the astronaut. In some of these designs some parts come trough stronger than others. Giving us some variation.
Besides character art, some work was also done on the main level. Mathis at your service!
To get a general overview of what our game will look like there's a clear need for the visuals of our main level. This week we got done with the midground of the level and a placeholder for the background. Seeming the game takes place in a prison in space I had to get a bit creative with how I'd make this clear using the mid and background.
After a little bit of itterating, the concept ended up like this:
After this I got started with blocking it out in our level to give a clearer overview how this would look:
Once this was clear I went ahead and made the final models which left us with this:
Now a little bit of texturing later left us with this:
For the final mid ground however I was still missing some subtle trim sheets, some fog and any other details to set the mood even better.
A bit of time later and voila, the final piece:
If time allows it I'd love to put even more time into this, but for now this is where we're at. A very solid base that clearly represents the conditions our brave inmates have been living in.
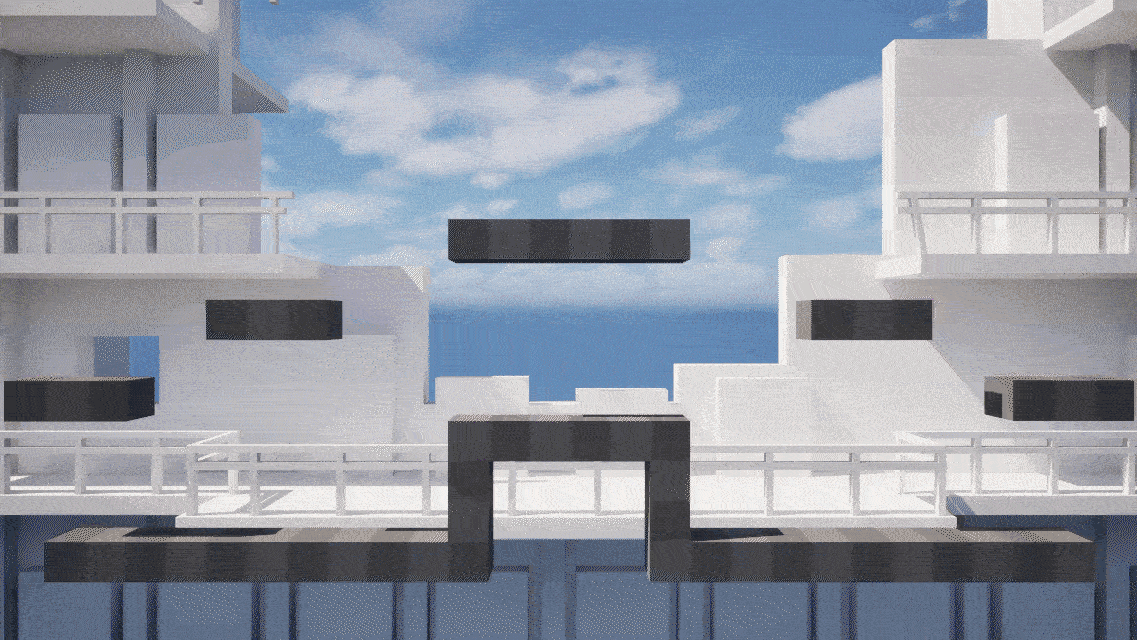
Technical
Dylan
I hope you enjoyed what the artists had to show because I sure did!
On the technical side, this week was all about getting the core systems in place. Since we started fresh, the focus was on building everything the right way now that we have clear answers from our prototyping phase.
- Jumping
- Shooting
- Basic Weapon Framework
- Basic Bullets
- Player Health
- Basic Weapon Framework
- Movement
- Basic Level
- Basic Character
The result? A T-posing character running around and shooting, not much to look at yet, but it gives us a solid foundation to build on.
What’s Coming Next?
Next week, we’ll start layering on more features, including:
- Dashing
- Weapon Pickup & Throwing
- Aiming
- And much more!
Closing Thoughts
This week was a slower one, but for good reason. Setting up the project properly and planning the codebase correctly takes time but now that the groundwork is done, we can fully focus on expanding the game!
By next week, we should be back to where our prototype left off but this time, with cleaner, structured code that’s ready for production.
That wraps up this week’s devlog! If you’re here for more technical details, stick around below. Otherwise see you next week!
Technical Deep-Dive
By now, you know how this goes. This time around, we are strictly following the guidelines established in our technical document, meaning no more AI-generated code. Let's start with a quick overview of what we tackled this week:
- Jumping
- Shooting
- Basic Weapon Framework
- Basic Bullets
- Player Health
- Basic Weapon Framework
- Movement
- Basic Level
- Basic Character
I’ll skip over the level, character, and bullet creation and focus on the good stuff.
Movement
For controls, I decided to use an event-based system to keep the code clean, well-structured, and properly decoupled. This ensures:
- The controller does not need to know who the player is.
- The player does not need to know who the controller is.
The controller simply sends an event when a button is pressed, without worrying about what happens next. The player listens for these events and executes the appropriate logic. No unnecessary dependencies between them.
Controller Implementation:
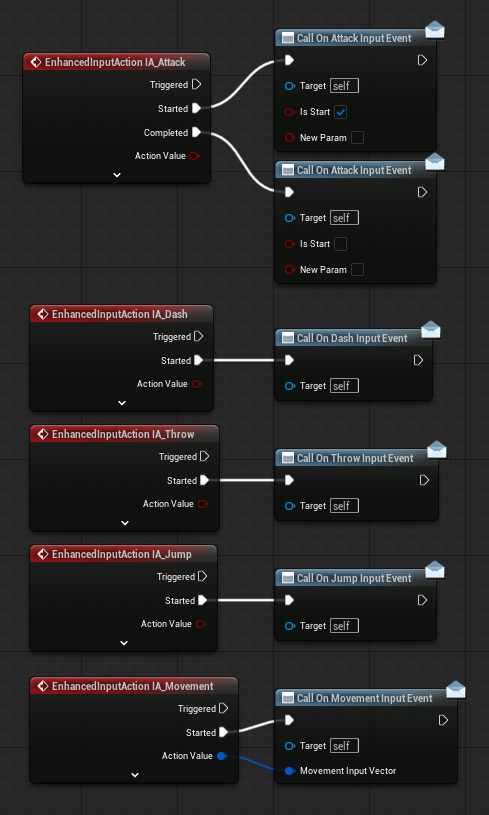
Player Implementation:
With controls set up, all that was left was to create the movement logic in C++ and call it in the appropriate custom event in Blueprint.
As expected, the movement is straightforward since Unreal Engine’s built-in character movement system provides everything we need with customizable parameters.
void APlayerCharacter::MovePlayer(const FVector2D& movement) { const FVector movementVector = FVector(movement.X, 0.0f, 0.0f).GetClampedToMaxSize(1.0f); AddMovementInput(movementVector); }
Simple, efficient, and effective.
Weapon Framework & shooting
Before shooting, we first need weapons. Here’s the structure I set up:
Weapon Hierarchy:
The Base Weapon Class contains all common properties across all weapons such as mesh, damage, knockback, and attack functions.
From there, we have three specialized subclasses for different weapon types:
- Automatic Weapons (e.g., assault rifles, SMGs)
- Semi-Automatic Weapons (e.g., pistols, shotguns)
- Melee Weapons (not yet implemented)
Each subclass has its own attack logic. Instead of managing multiple cases in a single function, I structured the framework so that the Attacking
function is pure virtual in the base class. Each subclass implements its own attack behavior.
Weapons with unique mechanics (such as the Thunder Gun) will have their own C++ class derived from the appropriate subclass (Semi-Automatic).
Pure Virtual functions in unreal
While working on the weapon structure, my initial idea was to make the BaseWeapon
class an abstract class with pure virtual functions. However, it turns out that pure virtual abstract classes are not fully supported in Unreal.
Key Takeaways:
-
Abstract Classes in Unreal:
- Unreal allows marking a class as abstract using
UCLASS(Abstract)
. - However, this only prevents the class from being inherited by a Blueprint.
- It does not behave like a true abstract class in standard C++.
- Unreal allows marking a class as abstract using
-
The Problem with Pure Virtual Functions:
- Unreal does not allow pure virtual functions in standard UCLASS-based classes.
- This is because Unreal requires every class to have at least one instance created during compilation.
- If a class has a pure virtual function, Unreal cannot generate an instance, leading to compilation errors.
- The common error message is:
'ABaseWeapon': cannot instantiate abstract class
-
The Solution: Using
PURE_VIRTUAL
- Unreal provides a macro called
PURE_VIRTUAL
to simulate pure virtual behavior. - Unlike standard C++, this does not enforce a compile-time error if the function is not overridden.
- Instead, it results in a runtime error if a child class fails to implement it.
- Unreal provides a macro called
Example usage:
virtual void FireWeapon(const bool isStart, APlayerCharacter* playerCharacter) PURE_VIRTUAL(ABaseWeapon::FireWeapon);
This approach allows us to maintain the structure of pure virtual functions while still adhering to Unreal’s compilation requirements. The more you know!
Shooting Logic:
Shooting works through two functions:
FireWeapon
– Determines when the attack happens.HandleAttack
– Handles projectile spawning and/or the attack swing for the melee.
Automatic vs. Semi-Automatic Firing:
- Automatic weapons continuously spawn projectiles while the fire button is held, at a rate determined by
m_rate_of_fire
. - Semi-automatic weapons use a boolean flag and an Unreal timer to enforce a delay between shots.
The melee attack system has not been implemented yet, as it will require animation-based hit detection and m_rate_of_fire
.
Handling Projectile Spawning
The HandleAttack
function spawns the projectile defined in the base class.
This logic is carried over from the prototype. Rotation adjustments are not yet implemented, as we don’t have aiming functionality.
To prevent self-collisions (since we can’t use tags as the players will have the same tags), we pass a reference to the player when spawning projectiles. This reference will likely also be used for aim direction later. Not ideal but I'm short on ideas for this one.
Adding New Weapons
To create a new weapon, we simply:
- Create a Blueprint inheriting from the correct base class.
- Adjust the weapon’s values (damage, fire rate, etc.).
For complex weapons, a new C++ class is required. This is how we created our first functional AK-47 prototype.
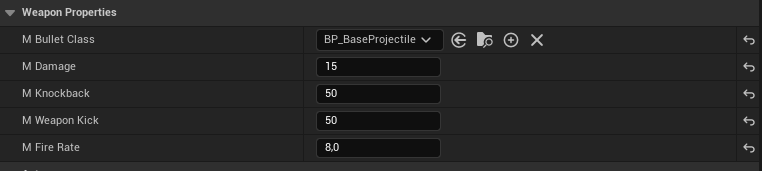
Player Health System
Like in the prototype, the health system is implemented as a component that can be attached to any character.
The DoDamage
function is defined in an interface rather than directly in the health component. While not strictly necessary, this allows for greater flexibility such as applying damage over time or affecting all players at once (e.g., chaos cards) should we need it later.
The health component manages the player’s health and triggers events upon death.
Final Words
That wraps up this week’s devlog.
I hope you enjoyed this deep dive. By next week, we’ll have more polished work to showcase. If you have any questions, comments, or suggestions, let us know!
See you next week!
Files
Get [Group26]Brawlstorm
[Group26]Brawlstorm
Status | In development |
Authors | Diji69, SamDK, Mkyv2210, InquisitiveOctopus, alessandromanzini |
Genre | Fighting |
Languages | English |
More posts
- Devlog 5: Square 2?25 days ago
- Devlog 3: Game Demo!39 days ago
- Devlog 2: Playable Prototype46 days ago
- Devlog 1.2: Aiming & Ragdolling53 days ago
- Devlog 1.1: Item catch prototype53 days ago
- Devlog 1: Research & Prototype54 days ago
Leave a comment
Log in with itch.io to leave a comment.